Our First Experience with Swift
Every other month or so we like to do a small Hackathon at 9elements. Last week, after months of hard client work, we finally had the chance to have one again.
During the two-day long event, several teams gather to explore new technologies, techniques, tools and build something for fun. After Apple announced their new programming language at WWDC 2014 earlier this June, we were excited to explore it at our next Hackathon.
DOAR
At our last Hackathon, we created a small app called DOAR, a door opener based on Arduino. DOAR is connected to our LAN and provides a simple API to open the door to our office. We’ve now extended DOAR so that it broadcasts the door ring to connected clients. Clients establish a WebSocket to DOAR. When the door rings, DOAR broadcasts a message to all connected clients. Clients use their WebSocket to send “open door’ commands to DOAR.
At this Hackathon, we created an OS X application with Xcode 6 (Beta) and Swift. The application opens a WebSocket to DOAR. When it receives a door bell message through the socket, it shows a notification in the Notification Center. You can directly interact with the notification to open the application and issue the “open door’ command. DOAR immediately sends a “did open door’ command to connected clients when it receives the first “open door’ command. This allows the OS X application to remove the notification from Notification Center so that it gets out of the user’s way.
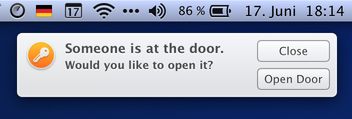
Swift
The DOAR client application for OS X was implemented entirely with Swift (and Interface Builder). The following paragraphs give an overview of things that we had not known or read/heard about before.
Access Control
Swift currently has no support for access control modifiers like @public
, @private
, and @protected
in Objective-C. Apple will deliver support for access modifiers in the final release of Xcode 6 in fall 2014 [1].
Selectors
When registering for notifications with a particular name via NSNotificationCenter, you typically provide a selector that gets called when some other part of the application posts a notification with that name. An Objective-C selector in Swift is a String.
swiftNSNotificationCenter.defaultCenter()
.addObserver(
self,
selector: "connectionDidReceiveDoorRing:",
name: ConnectionDidReceiveDoorRingNotification,
object: connection)
When initially writing the previous code, we forgot to include the colon in the selector. This caused a crash at the call site (when another part of the code posts that notification).
swiftNSNotificationCenter.defaultCenter()
.postNotificationName(
ConnectionDidReceiveDoorRingNotification,
object: self)
This makes sense, since the implementation of NSNotificationCenter synchronously invokes observers of that notification. However, the point at which the debugger stops in Xcode (at the exception breakpoint) and the debug output do not really indicate that the issue is a nonexistent selector in the observer.
Strings
In Swift string parameters are passed by value. If a call site provides a String
as the parameter to a function, Swift copies the complete String
into the parameter. This is in stark contrast to Objective-C where NSString
is passed by reference.
NSTimer
Usually, all Objective-C classes either directly or indirectly inherit from NSObject
. But Swift classes may not have a base class at all. It is perfectly fine to implement a Swift class that has no superclass.
When using NSTimer
in Swift code the target must inherit from NSObject
, otherwise the timer is not able to invoke the selector on the object.
Conclusion
We think that Swift is a well-engineered programming language with a big potential. It needs time to master a programming langue, but since we started coding in Swift we feel delighted by the simple and elegant code it allows us to write compared to Objective-C.
We are very excited about Swift and look forward to the final release this fall. In fact, we are excited enough that we created Swift Weekly, a weekly newsletter with the most interesting links to blog articles, code, and other stuff about Swift. Subscribe at swiftweekly.com or follow @swift_weekly on Twitter.