On the Future of Web Development
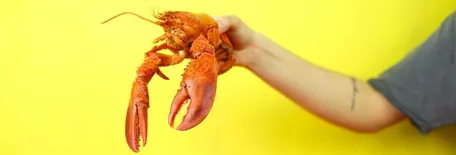
Rust is a strongly typed, low-level programming language built for safety, speed, and concurrency from the ground up. It is mostly developed by Mozilla and has been battle tested by over a hundred companies including Dropbox and the game studio Chucklefish. Mozilla’s new Quantum browser engine is in huge parts written in Rust and embedded in Firefox which is already used by millions of users every day. In this post, I would like to show JavaScript developers the advantages of working with Rust.
“It wasn’t always so clear, but the Rust programming language is fundamentally about empowerment: no matter what kind of code you are writing now, Rust empowers you to reach farther, to program with confidence in a wider variety of domains than you did before.”
- The Rust programming language
Feel the Empowerment
Although Rust is considered a low-level programming language, it doesn’t always feel that way. While most people connect strongly-typed languages with a lot of type annotations and much more verbose code, with Rust, you can relax and let the compiler’s type inference handle most of the work. Sometimes this makes Rust feel like a dynamically typed language.
rustfn main() {
print_whatever("hello");
print_whatever(134123);
print_whatever(true);
}
fn print_whatever<T: ToString>(input: T) {
let input: String = input.to_string();
println!("{}", input);
}
A method that is using a Trait for a type to make the interface more usable
Its trait system helps building abstractions over complex types that come at no runtime cost. A trait is a kind of interface with associated methods, types, and constants.
rust//! An example for the definition and implementation of traits
/// This is a basic trait. It defines a constant and a method to be
/// implemented by the concrete type
trait Eat {
const NAME: &'static str;
fn eat(&self) -> u32;
}
/// This is a struct. Who doesn't love bacon :)
struct Bacon {
calories: u32
}
impl Bacon {
pub fn new() -> Bacon {
Bacon {
calories: 9001
}
}
}
// Here we implement the Eat trait for our Bacon struct
impl Eat for Bacon {
const NAME: &'static str = "bacon";
fn eat(&self) -> u32 {
self.calories
}
}
/// This function takes an argument of "something that implements the Eat trait".
/// Then it prints the name and the calories of the argument
fn consume<T: Eat>(food: T) {
let name = T::NAME;
let calories = food.eat();
println!("Consumed {} and got {} calories in total", name, calories);
}
fn main() {
// Now we just create some bacon...
let bacon = Bacon::new();
// ... and consume it :)
consume(bacon);
}
For even more language magic there are macros. Rust’s macro system allows for slick DSLs while keeping the safety and speed of the language. For example, the method to print a line is a macro rather than a method. There is also a macro that makes it very easy to build a Vector of any kind:
rustfn main() {
let buf = vec![
"I",
"love",
"Rust",
"so",
"much"
];
let sentence: String = buf.join(" ");
}
Why should this be of interest for JavaScript developers?
Fewer runtime errors. Since Rust’s language model is rigorous, the compiler is able to detect most of the possible runtime errors. This may slow you down at first, but, after a while, it’ll make you as productive as you’ve never been before. In JavaScript, especially when you build large scale applications, you will write a lot of code that ensures type safety or does other sanity checks (often through unit tests). In Rust, most of these checks are intrinsic to the language.
WebAssembly support. The Rust team is currently working hard on a neat way to bring WebAssembly and Rust closer together. This includes a generator for bindings as well as a toolkit for publishing Rust crates on npm. For JavaScript developers that means that running Rust in the browser will be a fairly simple process in the future.
Webpack does it. Webpack 4 landed the initial support for WebAssembly and so emerged a loader for using Rust files as native ECMAScript modules. This combination of technologies helps to speed up your existing code. Thus, integrating Rust into existing JavaScript projects works seamlessly if you are using Webpack already.
There is a standard library. The project stdweb tries very hard and mostly succeeds in being the go-to standard library for client-side Rust on the Web. It makes it possible to call existing JavaScript libraries and makes JavaScript a first-class-citizen in Rust. Using cargo-web, an extension to cargo, it is a charm to write client-side WebApps in Rust. So, for JavaScript developers there are almost no elements missing for writing frontend applications.
Meet Yew. Yew is a modern Rust framework inspired by Elm and ReactJS. It lets you write powerful frontend web apps in Rust that run on WebAsssembly. It also provides a macro for writing components in a JSX-like syntax. This is also a great example for Rust’s powerful macro system.
rusthtml! {
<section class="todoapp",>
<header class="header",>
<h1>{ "todos" }</h1>
{ view_input(&model) }
</header>
<section class="main",>
<input class="toggle-all",
type="checkbox",
checked=model.is_all_completed(),
onclick=|_| Msg::ToggleAll, />
{ view_entries(&model) }
</section>
</section>
}
Yew’s JSX-like html macro for creating a component
Harness the ecosystem
Rust has a vast ecosystem that keeps growing. Despite its growth, the ecosystem is very stable because it is driven by a committed community. In fact, an integral part to Rust’s development is its openness. Every step of the development is publicly discussed and documented so everyone can take a look behind the curtains.
You know npmjs.com? Meet crates.io. Libraries in Rust are called crates. Just like npm packages you can install them when they offer a binary or add them as a dependency to your project and use them as a library. This part of the ecosystem is open as well. The website that powers crates.io as well as the package index are openly accessible on GitHub. Rust’s package manager cargo is built with due regard to a mixture of lessons learned from previous package managers as well as the works of Yehuda Katz, the creator of bundler. It is the central tool in the Rust world.
Documentation on-board. Rust comes with a built-in tool for generating documentation from Rust sources. The platform docs.rs automatically produces docs for existing crates and makes them searchable, so you get automatic documentation for almost every crate. You can find the official documentation for Rust on doc.rust-lang.org.
Keep track of your dependencies. If you are maintaining a huge crate with a lot of external dependencies, deps.rs helps you to keep track of all of them.
Deep-dive into the community
The community around Rust is incredible. The subreddit and the IRC channel (#rust on Mozilla IRC) are full of very helpful Rust developers of all tiers. There are tons of bloggers who write about their experiences with Rust. There are always so many things to do inside the Rust project, and everyone can join in and help develop future versions. Conferences like the RustFest in Europe and the RustConf in the US help to connect like-minded developers with similar levels of knowledge and help to spread the spirit of the language further. There are also many, many Meetups. Just check meetup.com for a Rust group close by.
Final thoughts
Learning Rust is very different from learning JavaScript as most information for learning Rust is right at your fingertips while resources for JavaScript are spread across many decoupled platforms. If you want to use Rust right now click through to rust-lang.org and install it. The official website holds all the information you need to start coding. If you don’t want to install Rust, you can try it online on play.rust-lang.org. I don’t think you should drop JavaScript altogether (at least not yet), but Rust is a language that might be omnipresent in the distant future of the web.
Getting more familiar with the language is a solid investment. For a good starting point on Rust and WebAssembly take a look at this template project: https://github.com/rustwasm/rust_wasm_template.
If you’d like to work with Rust in a professional capacity, shoot us an email at: contact@9elements.com and let’s get to know each other!
If you want to talk to me about Rust, you can send me a toot on Mastodon: https://ruhr.social/@sphinxc0re